Rather than starting with Swift, let’s start with the person who is designer/creator/maker of Swift programming language, Chris Lattner. The powerful LLVM (Low Level Virtual Machine) compiler that Apple is using with XCode to build Objective-C programs was developed by Chris. After the success of LLVM, Apple invests Chris for designing a new programming language; Chris and his team spend 4 years developing Swift.
Developer’s Viewpoint on Swift Programming Language
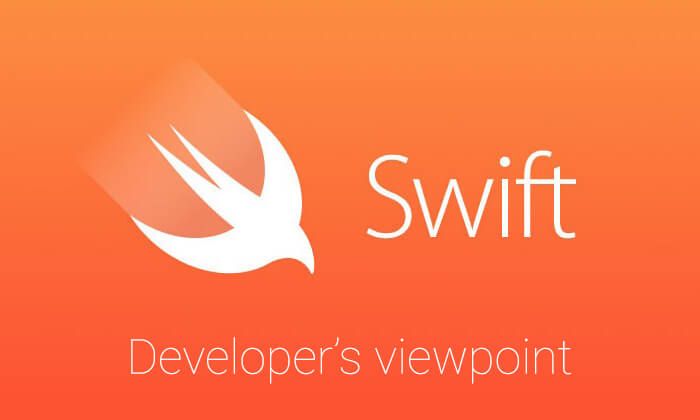
Why Apple Developed a New Programming Language?
This question will only knock into head of those persons who are not aware with Objective-C programming language.
When I started my carrier as an iOS developer, I was aware with Java and some other programming language, so initially comparison of Objective-C with other languages automatically comes into mind while developing iOS Applications, but later I used to deal with hurdles and limitations of Objective-C.
In Objective-C, you always have to write more lines of code as compared to Java, for the same functionality. For iOS developers, a big headache is memory management with Objective-C (Initially ARC was not available, it was introduced by Apple with iOS 5). Currently ARC is available for memory management, but still, it’s not accurate. You can’t implement accurate automatic garbage collection in a language that has pointers. You always need to take care of every single object with Objective-C; you didn’t get much time to focus on other parts of your application. Swift is fast, easy to learn and modern programming language; you did not need to waste your time with all this kind of issues in Swift. So, Apple has taken a right decision by introducing new programming language “Swift”.
Why Swift is Fast, Easy to Learn and Modern Programming Language?
Let’s discus the functionality of Swift to understand why it is easy, modern and fast programming language.
Easy:
Constants & Variables
Defining constants or variables is simple in Swift, use “let” to define constant and “var” to define variable.
let companyName = "XYZ" var stockPrice = 256
Type Inference
With Swift, you do not require to specify the type with constants and variables. The Compiler automatically inferred it based on the value you have assigned.
let userName = "Eric" // inferred as String let userAge = 28 // inferred as Int
String Interpolation & Mutability
For concatenating string in Swift is just a simple way to include value in string using \().
let Points = 500 let output = "Jimmy today you earned \(Points) points."
String Interpolation/Concatenation is a bit complex in Objective-C as compare to Swift. Here is the Objective-C code for the same:
int Points = 500; NSString *output = [NSString stringWithFormat:@"Jimmy today you earned %d points.",Points];
Check the simple way of string Mutability with swift:
var message = "Good Morning" message += "Karan" // Output will be "Good Morning Karan"
Optional variable & function return Types
In Swift, you can define possibly missing or optional value in variables, so you don’t need to worry about nil value exception, which you faced many times with Objective-C.
var optionValue: Int?
You can also make your function return type options, which is not possible to do with Objective-C.
func getUserName(userId: Int) -> String?
Array & Dictionary
Objective-C provides two types of all its variables, normal & mutable (Ex. NSArray, NSMutableArray, NSDictionary, NSMutableDictionary), I don’t know why Objective-C make all this bullshit, why it didn’t provide single type for all variable? Thanks to Swift, it makes easier to work with Array & Dictionary:
let usersArray = ["John","Duke","Panther","Larry"] // Array println(usersArray [0]) let totalUsers = ["male":6, "female":5] // Dictionary println(totalUsers["male"])
For-In: Ranges
Defining range in the loop is interesting with Swift, just use .. to make range that exclude the upper value, or use … to include the upper value in the range.
for i in 0...3 { println("Index Value Is \(i)") }
Modern:
Switch Cases
With Swift, now it’s possible to compare any kind of data with Switches (This is not possible to do with Objective-C). You can also make conditions with Case statement.
let user = "John Deep" switch user { case "John": let output = "User name is John." case let x where x.hasSuffix("Deep"): let output = "Surname of user is \(x)." default: let vegetableComment = "User not found."}
Functions
Swift provided some useful functionality with function. You can return multiple values from the function using tuple:
func getUserList() -> (String,String,String){ return ("John","Duke","Panther") }
In function, you can also pass variable number of arguments, collecting them into an array:
func addition(values:Int...) -> Int{ var total = 0 for value in values { total += value } return total }
You can set the default parameter value into a function:
func sayHello(name: String = "Hiren"){ println("Hello \(name)") }
Enumerations
Enumerations in Swift are not just for cases; it’s providing multiple functionalities. Like classes, now you can also write methods
into enumerations: enum Section: Int { case First = 1 case Second, Third, Fourth case Fifth func Message() -> String { return "You are into wrong section" } }
Closures
Objective-C providing blocks, small chunks of code that can be passed around within an application. It is very useful to execute specific task on some user action. It is convenient and also improves readability of code. So I am not surprised that blocks are back into the Swift, with name Closures. In Swift, you can write Closures without a name with braces ({}).
var users = ["John","Duke","Panther","Larry"] users.sort({(a: String, b: String) -> Bool in return a < b }) println(users) // Output = ["Duke", "John", "Larry", "Panther"]
Tuples
Swift introduced advanced types, Tuples. It’s not available in Objective-C. Tuples enable you to create and pass groupings of values. As we have seen in “functions”, tuples allow you to return multiple values as a single compound value.
(404, "Page Not Found", 10.5) // (Int, String, Double)
Generics
You can make generics of any functions, classes or enumerations when you required variety of variable types. Ex. You can create generic function that uses content of the array, regardless of what kinds of values stored in the array. To make a generic, just write a name inside angle brackets:
enum jobType<T>{ case None case Some(T) }
Structs
Structs are similar to classes, but it excludes inheritance, deinitializers and reference counting. The Main difference between classes & structures is that classes are passed by reference, and structures are always passed around by copying them.
struct normalStructure { func message(userName: String) -> String{ return "Hello \(userName)" } }
Fast:
Auto memory management
- ARC has been already available in Objective-C, but it’s working more accurately with Swift. ARC track and manage your app memory usage, so you did not require managing memory yourself. ARC frees up your created objects when they are no longer needed.
- In Swift you do not require to specify strong or week property with objects to tell ARC that which object you want to hold temporarily and which for long use, ARC automatically identify it by reference of every object.
LLVM compiler
- The LLVM compiler converts Swift code into native code. They optimized LLVM compiler for performance. Using LLVM, Apple makes it possible to run Swift code side-by-side with Objective-C.
- LLVM is currently the core technology of Apple developer tools; it helps to get the most out of iPhone, iPad and Mac hardware.
Playgrounds
- For me, using Playgrounds is like playing games with my code. Playgrounds make writing Swift code simple & fast.
- Playgrounds help you to view your variables into a graph, it directly displays output of your code, and you do not require to compile it every time. It also displays animated scene of your SpriteKit game.
- Playground is like a testing tool of your code. First check output of your code with playground, when you have perfected it, just move it into your project.
- Playground will be more useful when you are designing any new algorithm or experimenting with some new APIs.