In this article, you’ll learn all about JavaScript arrow functions and regular functions and what is the major difference between them and when we should use one over the other. You’ll see lots of examples that illustrate how they work.
How and Where We use Arrow Function and Regular Function?
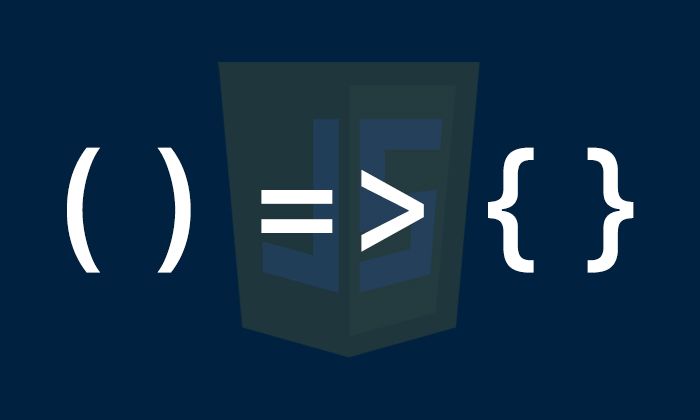
1. Syntax Difference
Functions are like recipes where you store useful instructions to accomplish something you need to happen in your program, like performing an action or returning a value. By calling your function, you execute the steps included in your recipe. You can do so every time you call that function without needing to rewrite the recipe again and again.
It is important to understand how the arrow function behaves differently compared to the regular ES5 functions.
There is some difference in syntax structure of arrow function and regular function. Let’s check it out.
a) Regular Function
Here’s the standard way of declaration of a regular function and calling that function in Javascript.
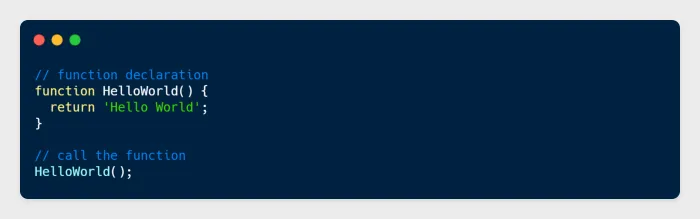
You can also write the same function as a function expression, like this-
let HelloWorld = function () {
return 'Hello World';
}
b) Arrow Function
JavaScript arrow functions are also called Fat arrow functions. These were introduced in ES6. Their concise syntax and handling of the this
keyword has made JavaScript arrow functions an ES6 favourite among developers.
An arrow function can be defined in multiple ways as described below:
i) No Parameter
If there are no parameters, then you can place empty parentheses before =>
like the below example.
() => 'Hello';
You can even skip even parenthesis:
_ => 'Hello';
ii) Single Parameter
If there’s only one parameter then syntax will be written as two types given below.
iii) Multiple Parameter
If there is more than one parameter available then it will be written as-
let add = (x, y) => x + y;
iv) Statements (as opposed to expressions)
Multiple parameters require parentheses. Multiline statements require body braces and return.
v) Block body
If your function is in a block, you must also use the return
keyword for returning a value.
let add = (x, y) => {
let z = 10;
return x + y + z;
}
vi) Object Literals
If you are returning an object then you need to wrap it in parentheses. This forces the interpreter to evaluate what is inside the parentheses, and the object literal is returned.
let candidate = a =>({ age: 24 })
2. Syntactically Anonymous
It is important to note that arrow functions are anonymous, which means that they are not named. This will create some issues listed below.
a) Harder to debug
When you get an error, you will not be able to trace the name of the function or the exact line number where it occurred.
b) No self-Referencing
If your function needs to have a self-reference at any point (e.g. recursion, an event handler that needs to unbind), it will not work.
3. Use of “new”
keyword
a) Regular Function
Regular functions are constructible, so they can be called using the new
keyword.
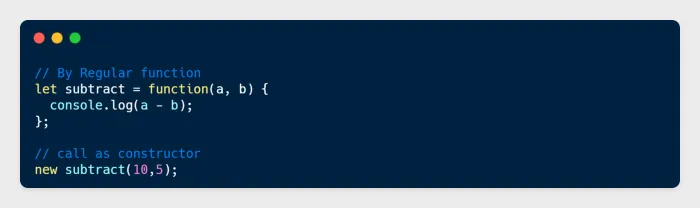
b) Arrow Function
On the other hand, Arrow functions are not constructible so the arrow function will never use as a constructor. That’s why arrow functions are never used with new
keyword.
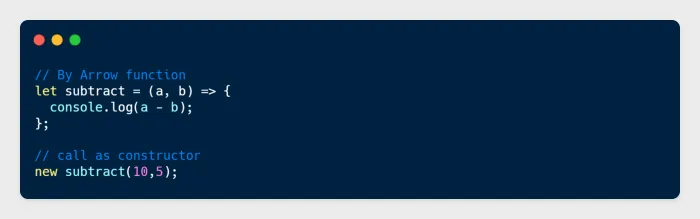
4. Use of “this” keyword
a) Regular Function
We can use this
keyword in regular function and we can access all the variables and functions using this
.
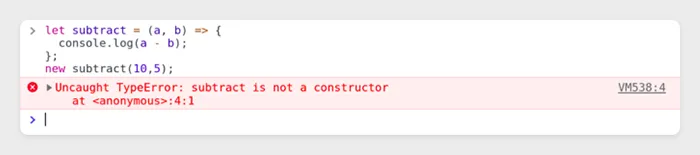
b) Arrow Function
But on the other hand, we can not use this
keyword directly into arrow function because it does not contain its own this
. So when we use this
keyword in the arrow function, it will return an undefined value.
So to resolve this issue we need to bind this
keyword into this arrow function like below.
5. Instantiating a function
We create an empty object inside a function and then we add some properties and functions into that object and then we return that object.
Every time the function is called we will have access to the methods that were created. Here is an example of functional instantiation:
6. Passing Arguments
a) Regular Function
b) Arrow Function
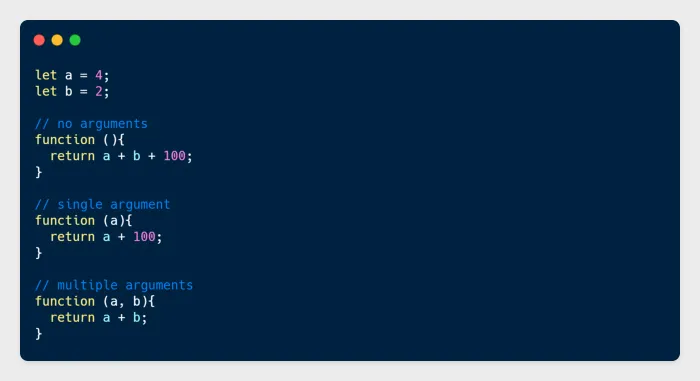
7. Returning Values
a) Regular Function
return
key is used to return value or statement in the regular functions as given below.
But if the return statement is missing or there is no expression after return statement then the regular function returns undefined value.
b) Arrow Function
We can return values from the arrow function the same way as from a regular function, but with one useful exception.
If the function contains only one expression then we can return value without using return
key as given below-
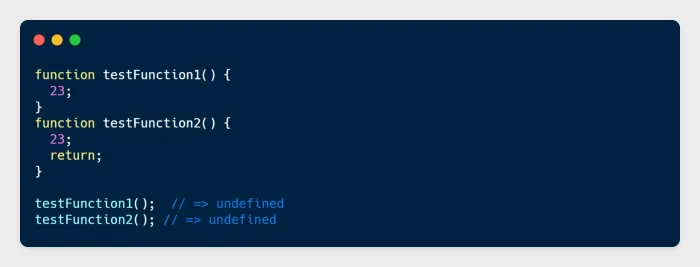
But if we have multiple expression in arrow function then we should use return
key for returning value as given below-
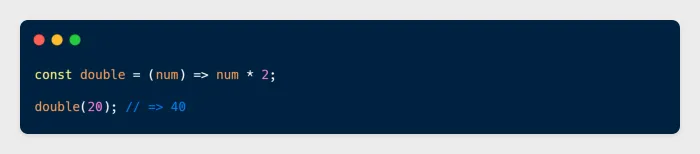
8. Methods
a) Regular Function
We can check from the following example in which PrintUserName()
is defined in class User
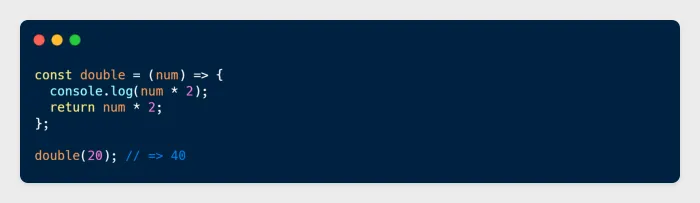
But when we try to use the method as a callback for example to setTimeout()
or an event listener. In such cases, you might encounter difficulties accessing this
value.
Let’s see the example where we use PrintUserName()
the method as callback insetTimeout().
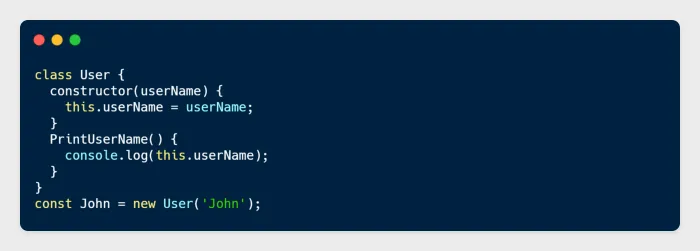
After 1 second, undefined
is logged to the console. To overcome this issue we have to bind this
value as given below-

Binding this
manually requires boilerplate code, especially if you have lots of methods. There’s a better way: the arrow functions as a class field.
b) Arrow Function
Now, in contrast with regular functions, the method defined using an arrow binds this
lexically to the class instance.
We can use PrintUserName()
arrow function as a method inside class User
.

Now we can use John.PrintUserName
as a callback without any manual binding of this
. The value of this
inside PrintUserName()
the method is always the class instance:
Final Comments
It is not that arrow functions are always a good choice in all scenarios. Please use them wisely.