7. Create Wrappers
There are many beautiful UI libraries available for you to pick up.
We highly suggest you create wrapper components around the components offered by the UI libraries.
For example, if your application has a lot of input controls, please create a wrapper around the input control of the library and use that instead.
This will allow you to add more functionality on top of what you are already getting.
In the below example, we’re using Ant Design’s Image component inside the wrapper called CBImage and we use this wrapper wherever we need the Image component. You can call CBImage as a widget.
Just prefix any widget/wrapper you create with your product’s initial letters to avoid confusion with library components.
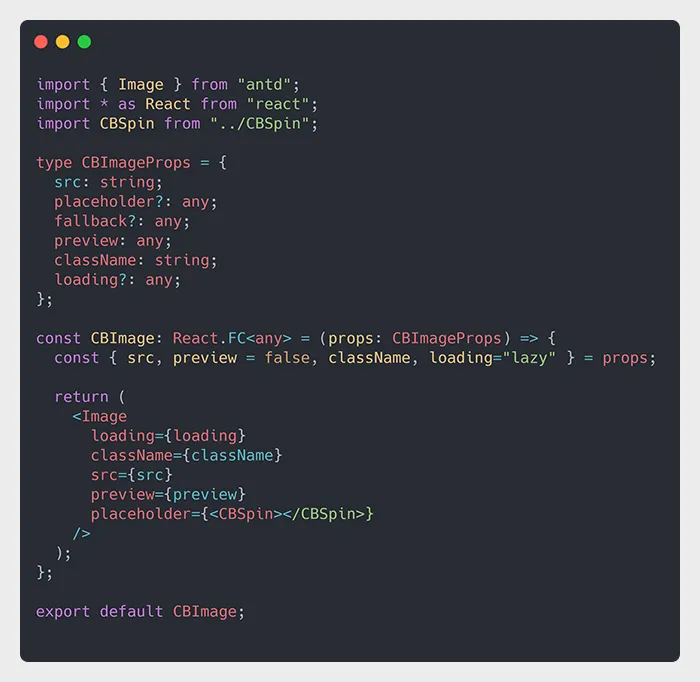
8. Write Transformation Layer
As a frontend developer, before starting your work on any story, you always discuss with the backend developer what the JSON structure of any object will be.
Well, that is a good practice, but what if the backend team is lagging behind in terms of development?
Will you sit idle for a few days? Of course not. What you can do is create your own View Models and start designing the application with test (or you can call it stub) data.
And when the time comes for integration with APIs, you just transform your model into the model that is expected by the backend.
The model that the frontend needs will be suffixed with VM and the model that the backend needs will be suffixed with DTO.
In the below example, you can see that the backend response is converted to what the frontend needs. It also offers you greater flexibility out of the box.
Let’s say the backend developer decides to change any of the properties, you just need to update this code and nothing else.
Your components would still work as if they don’t know any change happened.
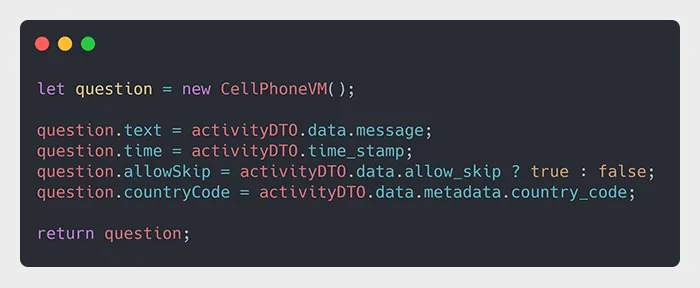
9. Focus on Maintainability
The best feature of modern frameworks is the components that you can reuse at multiple places.
But if that same component is given multiple responsibilities to handle, then you are misusing the component.
A component should be designed with one feature in mind. Bypassing multiple props to the component and using if-else conditions in the component to render different layouts is a bad idea.
Ideally, you are trying to save development hours in the beginning but as time passes that same component will start accommodating more and more features and very soon it will become a huge component.
Now let some months pass, and you come back to modify the component either due to a bug or to add a new feature.
Even though the code was written by you, it will take some time to understand what you had written long back.
Assuming your memory is good and you remember everything vividly, you start injecting additional code and testing the functionality.
But the time it took you to add new code would be longer this time and there are high chances that you might introduce new bugs.
The reason? This same component is used at multiple places and you might have forgotten to verify all the scenarios.
For example, if you are supposed to create a responsive site, and you need your header to look different on different devices, then it’s better to create two separate components.
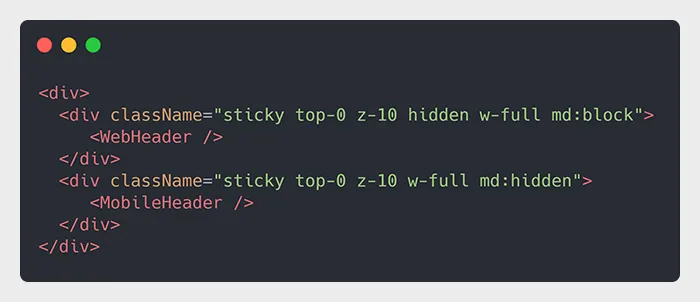
It is believed that if you don’t focus on maintainability from the beginning, your maintenance cost will be higher than the development.